Top 10 Data Science Projects For Beginners with PDF
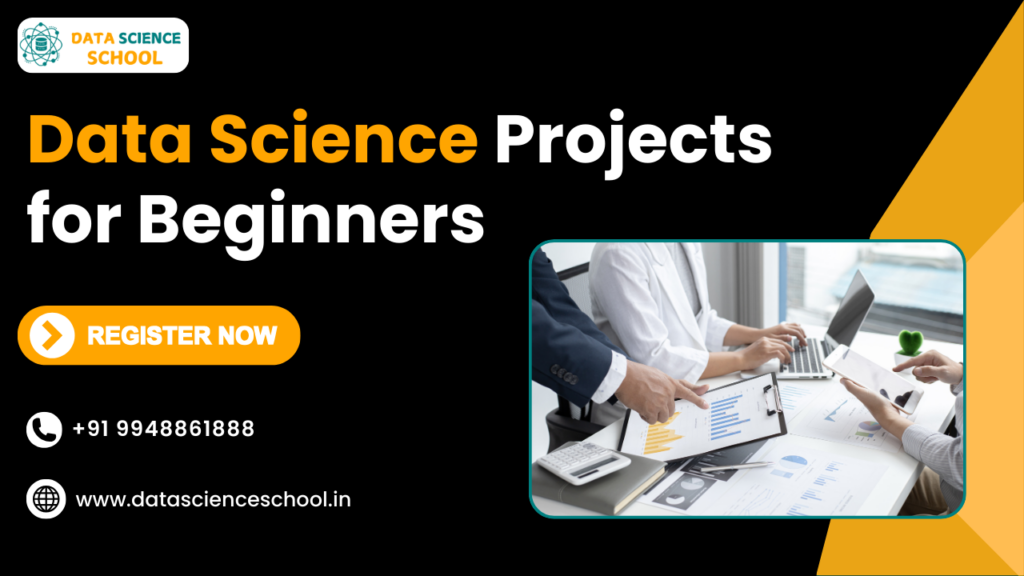
Get Data Science Projects for Syllabus PDF Download
Here you have to find the data science top 10 projects .we have clearly explained the total project insight ,like project description, skills required, steps to execute the project,expected outcomes.Hope this blog will help you regarding data science projects.please to read till end to get answer your questions.
Top 10 Data Science Projects For Beginners
Project 1:Fake News Detection Using Machine Learning Data Science
Project Description
This Data Science projects aims to identify fake news articles by applying machine learning methods. The objective is to categorize news articles as either fake or real by analyzing their textual content.The project involves preprocessing the text data, transforming it into numerical representations using techniques like TF-IDF, and applying machine learning models such as Logistic Regression or Naive Bayes. This hands-on project helps beginners understand text analysis, feature extraction, and classification methods, preparing them for real-world challenges in data science.
Skills Required
- Programming:
- Proficiency in Python.
- Proficiency in using libraries such as Pandas, NumPy, Scikit-learn, and NLTK for data manipulation and analysis.
- Text Preprocessing:
- Cleaning text (removing punctuation, stop words, and irrelevant characters).
- Tokenization and stemming.
- Machine Learning:
- Familiarity with classification algorithms like Logistic Regression, Naive Bayes, and Random Forest.
- Experience in model evaluation using metrics like accuracy, precision, recall, and F1-score.
- Data Visualization:
- Use tools like Matplotlib and Seaborn for plotting results and insights.
Steps to Execute the Project
Step 1: Data Collection
- Use publicly available datasets like the Fake News Dataset from Kaggle.
- Import the dataset into a Pandas DataFrame for further analysis.
- python
import pandas as pd
data = pd.read_csv(‘fake_news_dataset.csv’)
print(data.head())
Step 2: Data Preprocessing
- Clean the text data by removing special characters, numbers, and stop words.
- Tokenize the text and apply stemming or lemmatization.
python
from nltk.corpus import stopwords
from nltk.stem.porter import PorterStemmer
import re
from nltk.stem import PorterStemmer
from nltk.corpus import stopwords
import re
ps = PorterStemmer()
def clean_text(text):
# Remove non-alphabetical characters
text = re.sub(r'[^a-zA-Z]’, ‘ ‘, text)
# Perform stemming and filter out stopwords
text = [ps.stem(word) for word in text.split() if word.lower() not in set(stopwords.words(‘english’))]
return ‘ ‘.join(text)
data[‘cleaned_text’] = data[‘text’].apply(clean_text)
Step 3: Feature Extraction
- Convert text into numerical data using TF-IDF or CountVectorizer.
python
from sklearn.feature_extraction.text import TfidfVectorizer
tfidf = TfidfVectorizer(max_features=5000)
X = tfidf.fit_transform(data[‘cleaned_text’]).toarray()
y = data[‘label’] # Assuming ‘label’ column contains 0 for real and 1 for fake.
Step 4: Model Training
- Split the data into training and testing sets.
- Train a classification model like Logistic Regression.
python
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score, classification_report
# Splitting data into 80% training and 20% testing sets, with random state fixed for consistency
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = LogisticRegression()
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
Step 5: Model Evaluation
- Evaluate the model’s performance by calculating accuracy, precision, recall, and the F1-score
python
print(“Accuracy:”, accuracy_score(y_test, y_pred))
print(“Classification Report:\n”, classification_report(y_test, y_pred))
Step 6: Deployment
- Build a simple web interface using Flask or Streamlit for testing the model on new inputs.
python
# Example for Streamlit
import streamlit as st
st.title(“Fake News Detection”)
user_input = st.text_area(“Enter news text:”)
if st.button(“Check”):
user_vector = tfidf.transform([clean_text(user_input)]).toarray()
prediction = model.predict(user_vector)
result = “Fake News” if prediction[0] == 1 else “Authentic News”
st.write(f”The news is: {result}”)
Expected Outcomes
- A trained machine learning model capable of classifying news as fake or real with high accuracy.
- Hands-on experience in text preprocessing, feature engineering, and machine learning.
- A functional interface to test the model with real-world inputs.
- A deeper understanding of how machine learning can address misinformation challenges.
Project2:COVID-19 Data Analysis and Visualization with Data Science
Project Description
This Data Science project involves analyzing COVID-19 data to extract meaningful insights and create visualizations that highlight trends such as case growth, recovery rates, and vaccination progress. The project will use publicly available datasets and Python libraries to clean, process, and visualize the data. Key objectives include understanding how the pandemic spread over time, identifying patterns across regions, and presenting actionable insights through dashboards or visual reports.
Skills Required
- Data Analysis:
- Proficient in Python, with specialization in leveraging Pandas for effective data manipulation
- Data Cleaning:
- Handling missing values, duplicates, and formatting inconsistencies in large datasets.
- Visualization:
- Generating visualizations using libraries such as Matplotlib, Seaborn, and Plotly.
- Geospatial Analysis (Optional):
- Using tools like GeoPandas or Folium for mapping COVID-19 trends by region.
Steps to Execute the Project
Step 1: Data Collection
- Obtain datasets from sources like WHO or Kaggle.
python
import pandas as pd
url = “https://path-to-covid-dataset.csv” # Replace with an actual dataset URL
data = pd.read_csv(url)
print(data.head())
Step 2: Data Cleaning
- Handle missing or inconsistent data.
- Convert date columns to datetime format for time-series analysis.
python
data[‘Date’] = pd.to_datetime(data[‘Date’])
data.fillna(0, inplace=True) # Replace missing values with 0
Step 3: Exploratory Data Analysis (EDA)
- Analyze key metrics like total cases, recoveries, and deaths over time.
- Group data by region or country for regional analysis.
python
# Example: Total cases over time
total_cases = data.groupby(‘Date’)[‘Confirmed’].sum()
print(total_cases)
Step 4: Visualization
- Create time-series plots for confirmed cases, recoveries, and deaths.
- Use bar charts for country-wise comparisons and heatmaps for regional trends.
python
import matplotlib.pyplot as plt
# Time-series plot
plt.figure(figsize=(10, 6))
plt.plot(total_cases, label=’Total Cases’, color=’blue’)
plt.title(‘COVID-19 Cases Over Time’)
plt.xlabel(‘Date’)
plt.ylabel(‘Number of Cases’)
plt.legend()
plt.show()
Step 5: Advanced Visualization
- Use Plotly or Folium for interactive visualizations.
python
import plotly.express as px
# Interactive scatter plot
fig = px.scatter(data, x=’Date’, y=’Confirmed’, color=’Country’, title=”COVID-19 Confirmed Cases by Country”)
fig.show()
Step 6: Dashboard Creation (Optional)
- Build a dashboard using Streamlit or Tableau to make the visualizations interactive.
python
import streamlit as st
st.title(“COVID-19 Data Dashboard”)
st.line_chart(total_cases)
st.bar_chart(data.groupby(‘Country’)[‘Confirmed’].sum())
Expected Outcomes
- Clear visualizations showing trends in COVID-19 cases, recoveries, and deaths.
- Insights into regional and temporal patterns of the pandemic.
- Hands-on experience in data cleaning, analysis, and visualization using Python.
- An interactive dashboard for presenting the findings to a broader audience.
Project 3:Customer Churn Prediction in Telecom with Data Science
Project Description
The objective of this project is to forecast customer churn in the telecommunications sector by employing machine learning methods.The objective is to identify customers likely to discontinue a telecom service and understand the factors contributing to churn. By analyzing historical customer data, including demographics, usage patterns, and service-related attributes, we will build a predictive model to classify customers as churned or retained. The project provides hands-on experience in data preprocessing, feature engineering, and building classification models to address a real-world busin
Skills Required
- Programming Skills:
- Extensive experience in Python, with a strong command of libraries like Pandas, NumPy, and Scikit-learn.
- Data Cleaning and Preprocessing:
- Handling missing values, categorical data encoding, and feature scaling.
- Machine Learning:
- Understanding of classification models such as Logistic Regression, Random Forest, and Gradient Boosting.
- Model evaluation using metrics like confusion matrix, accuracy, precision, and recall.
- Data Visualization:
- Skilled in using Matplotlib and Seaborn to visualize trends in customer data.
Steps to Execute the Project
Step 1: Data Collection
- Use publicly available telecom datasets, such as the Telco Customer Churn dataset from Kaggle.
python
import pandas as pd
data = pd.read_csv(‘telco_customer_churn.csv’) # Replace with the actual dataset path
print(data.head())
Step 2: Data Cleaning and Preprocessing
- Manage missing values and encode categorical data using methods such as one-hot encoding or label encoding.
- Scale numerical features to normalize their range.
python
# Example: Encoding categorical variables
data[‘Churn’] = data[‘Churn’].map({‘Yes’: 1, ‘No’: 0}) # Target variable encoding
# Handle missing values
data.fillna(data.median(), inplace=True)
# Feature scaling
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
data[[‘MonthlyCharges’, ‘TotalCharges’]] = scaler.fit_transform(data[[‘MonthlyCharges’, ‘TotalCharges’]])
Step 3: Exploratory Data Analysis (EDA)
- Analyze trends like churn rate across demographics, contract type, and tenure.
- Visualize data distributions and correlations between features.
python
import seaborn as sns
import matplotlib.pyplot as plt
# Visualizing churn by contract type
sns.countplot(data=data, x=’Contract’, hue=’Churn’)
plt.title(‘Churn Rate by Contract Type’)
plt.show()
Step 4: Feature Selection
- Identify important features influencing churn using correlation analysis or feature importance scores.
python
# Correlation analysis
corr = data.corr()
sns.heatmap(corr, annot=True, cmap=’coolwarm’)
plt.title(‘Feature Correlation Matrix’)
plt.show()
Step 5: Model Training
- Split the dataset into training and testing sets.
- Train machine learning models like Logistic Regression or Random Forest for classification.
python
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import classification_report
X = data.drop([‘Churn’, ‘CustomerID’], axis=1) # Exclude target and non-relevant columns
y = data[‘Churn’]
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = RandomForestClassifier(random_state=42)
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
Step 6: Model Evaluation
- Evaluate a model’s effectiveness using indicators like accuracy, precision, recall, and F1-score.
python
print(“Classification Report:\n”, classification_report(y_test, y_pred))
Step 7: Deployment (Optional)
- Deploy the model using Streamlit or Flask to provide predictions for new customer data.
python
import streamlit as st
st.title(“Customer Churn Prediction”)
user_input = st.text_input(“Enter Customer Details (as comma-separated values):”)
if user_input:
input_data = [float(val) for val in user_input.split(‘,’)]
prediction = model.predict([input_data])
result = “Churned” if prediction[0] == 1 else “Retained”
st.write(f”The customer is likely to: {result}”)
Expected Outcomes
- A machine learning model capable of accurately predicting customer churn in the telecom industry.
- Insights into key factors contributing to customer churn.
- Hands-on experience in data preprocessing, model building, and evaluation.
- Potential for deployment as a tool to assist telecom companies in retaining customers.
Project 4:House Price Prediction with Advanced Regression Techniques In Data Science
Project Description
This project involves predicting house prices using advanced regression techniques. By analyzing datasets with features like location, size, number of bedrooms, and other attributes, we aim to build a predictive model that can estimate property prices accurately. The project emphasizes feature engineering, hyperparameter tuning, and the application of regression models such as Linear Regression, Decision Trees, and Gradient Boosting. This hands-on experience prepares you to tackle real-world regression problems with advanced tools and methodologies.
Skills Required
- Data Analysis:
- Proficiency in Python, with Pandas and NumPy for data handling.
- Data Preprocessing:
- Feature engineering and handling missing values.
- Encoding categorical variables and scaling numerical data.
- Regression Models:
- Knowledge of Linear Regression, Ridge, Lasso, Random Forest, and Gradient Boosting models.
- Model Tuning:
- Expertise in hyperparameter tuning using GridSearchCV or RandomizedSearchCV.
- Visualization:
- Proficiency in Matplotlib and Seaborn for creating insightful visualizations.
Steps to Execute the Project
Step 1: Data Collection
- Use datasets like the Ames Housing Dataset or the Boston Housing Dataset, available on platforms like Kaggle.
python
import pandas as pd
data = pd.read_csv(‘housing_data.csv’) # Replace with the actual dataset path
print(data.head())
Step 2: Data Cleaning and Preprocessing
- Handle missing values and outliers.
- Encode categorical variables and scale numerical features.
python
from sklearn.preprocessing import OneHotEncoder, StandardScaler
# Handling missing values
data.fillna(data.median(), inplace=True)
# Encoding categorical variables
encoder = OneHotEncoder(drop=’first’, sparse=False)
categorical_features = data.select_dtypes(include=’object’)
encoded_features = pd.DataFrame(encoder.fit_transform(categorical_features), columns=encoder.get_feature_names_out())
# Scaling numerical features
scaler = StandardScaler()
numerical_features = data.select_dtypes(include=[‘int64’, ‘float64’])
scaled_features = pd.DataFrame(scaler.fit_transform(numerical_features), columns=numerical_features.columns)
# Merging processed data
data_processed = pd.concat([scaled_features, encoded_features], axis=1)
Step 3: Exploratory Data Analysis (EDA)
- Analyze relationships between features and the target variable (house price).
- Visualize correlations using heatmaps and scatter plots.
python
import seaborn as sns
import matplotlib.pyplot as plt
# Heatmap of feature correlations
corr = data_processed.corr()
sns.heatmap(corr, annot=True, cmap=’coolwarm’)
plt.title(‘Feature Correlation Heatmap’)
plt.show()
# Scatter plot for specific features
sns.scatterplot(data=data, x=’LivingArea’, y=’Price’)
plt.title(‘Living Area vs. Price’)
plt.show()
Step 4: Model Selection
- Split the data into training and testing sets.
- Train multiple regression models for comparison.
python
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.ensemble import GradientBoostingRegressor
from sklearn.metrics import mean_squared_error
X = data_processed.drop(‘Price’, axis=1)
y = data_processed[‘Price’]
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Linear Regression
lr_model = LinearRegression()
lr_model.fit(X_train, y_train)
# Gradient Boosting Regressor
gb_model = GradientBoostingRegressor(random_state=42)
gb_model.fit(X_train, y_train)
Step 5: Model Evaluation
- Evaluate models using metrics like Mean Squared Error (MSE) and R-squared.
python
# Linear Regression evaluation
lr_predictions = lr_model.predict(X_test)
lr_mse = mean_squared_error(y_test, lr_predictions)
lr_r2 = lr_model.score(X_test, y_test)
# Gradient Boosting evaluation
gb_predictions = gb_model.predict(X_test)
gb_mse = mean_squared_error(y_test, gb_predictions)
gb_r2 = gb_model.score(X_test, y_test)
print(f”Linear Regression – MSE: {lr_mse}, R2: {lr_r2}”)
print(f”Gradient Boosting – MSE: {gb_mse}, R2: {gb_r2}”)
Step 6: Hyperparameter Tuning
- Optimize model performance using GridSearchCV or RandomizedSearchCV.
python
from sklearn.model_selection import GridSearchCV
# Hyperparameter tuning for Gradient Boosting
param_grid = {
‘n_estimators’: [100, 200, 300],
‘learning_rate’: [0.01, 0.1, 0.2],
‘max_depth’: [3, 5, 7]
}
grid_search = GridSearchCV(GradientBoostingRegressor(random_state=42), param_grid, scoring=’neg_mean_squared_error’, cv=3)
grid_search.fit(X_train, y_train)
best_model = grid_search.best_estimator_
print(f”Best Parameters: {grid_search.best_params_}”)
Step 7: Deployment (Optional)
- Deploy the model using Flask or Streamlit for predicting house prices based on user inputs.
python
import streamlit as st
st.title(“House Price Prediction”)
user_input = st.text_input(“Enter features (comma-separated):”)
if user_input:
input_data = [float(x) for x in user_input.split(‘,’)]
prediction = best_model.predict([input_data])
st.write(f”Predicted House Price: ${prediction[0]:,.2f}”)
Expected Outcomes
- An optimized regression model capable of accurately predicting house prices.
- Insights into key factors affecting property prices.
- Practical experience in advanced regression techniques and hyperparameter tuning.
- A functional interface or report for presenting predictions and findings.
Project 5:Traffic Flow Prediction Using Real-Time Data With Data Science
Project Description
This Data Science projects involves predicting traffic flow using real-time data from sensors or publicly available traffic datasets. The goal is to analyze factors influencing traffic patterns, such as time of day, weather conditions, and road types, to build a predictive model for traffic density. By utilizing advanced machine learning algorithms, the project offers a practical solution to manage traffic congestion and improve urban mobility. The output includes traffic predictions that can assist city planners, commuters, and logistics companies.
Skills Required
- Data Analysis:
- Proficiency in Python and libraries like Pandas and NumPy for handling and analyzing time-series data.
- Time-Series Forecasting:
- Knowledge of algorithms like ARIMA, LSTMs, or Prophet for traffic flow prediction.
- Real-Time Data Handling:
- Working with APIs or sensors to retrieve live traffic data.
- Visualization:
- Expertise in Matplotlib, Seaborn, and Plotly for data visualization.
- Machine Learning:
- Experience with regression and advanced ML models like Random Forest and Gradient Boosting.
Steps to Execute the Project
Step 1: Data Collection
- Use publicly available traffic datasets from sources like Google Maps APIs, OpenTraffic, or city transportation departments.
python
import requests
import pandas as pd
# Example: Fetching data from a traffic API
api_url = “https://example-traffic-api.com/data”
response = requests.get(api_url)
data = response.json()
traffic_data = pd.DataFrame(data)
print(traffic_data.head())
Step 2: Data Cleaning and Preprocessing
- Handle missing values, outliers, and normalize data.
- Convert time-related data into features like day, hour, and weekday.
python
traffic_data[‘timestamp’] = pd.to_datetime(traffic_data[‘timestamp’])
traffic_data[‘hour’] = traffic_data[‘timestamp’].dt.hour
traffic_data[‘day_of_week’] = traffic_data[‘timestamp’].dt.dayofweek
# Filling missing values
traffic_data.fillna(traffic_data.median(), inplace=True)
Step 3: Exploratory Data Analysis (EDA)
- Analyze traffic trends across hours, days, and weather conditions.
- Visualize correlations between variables.
python
import seaborn as sns
import matplotlib.pyplot as plt
# Traffic flow by hour
sns.lineplot(data=traffic_data, x=’hour’, y=’traffic_flow’)
plt.title(‘Traffic Flow by Hour’)
plt.show()
Step 4: Feature Engineering
- Create lagged features for time-series modeling (e.g., previous hour traffic).
- Add external factors like weather or events as features.
python
traffic_data[‘lag_1’] = traffic_data[‘traffic_flow’].shift(1)
traffic_data[‘rolling_avg’] = traffic_data[‘traffic_flow’].rolling(window=3).mean()
Step 5: Model Training
- Split data into training and testing sets.
- Train regression or time-series models like Random Forest, ARIMA, or LSTMs.
python
from sklearn.ensemble import RandomForestRegressor
from sklearn.model_selection import train_test_split
X = traffic_data[[‘hour’, ‘day_of_week’, ‘lag_1’, ‘rolling_avg’]]
y = traffic_data[‘traffic_flow’]
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = RandomForestRegressor(random_state=42)
model.fit(X_train, y_train)
Step 6: Model Evaluation
- Evaluate the model using metrics like Mean Absolute Error (MAE) or Mean Squared Error (MSE).
python
from sklearn.metrics import mean_absolute_error
y_pred = model.predict(X_test)
mae = mean_absolute_error(y_test, y_pred)
print(f”Mean Absolute Error: {mae}”)
Step 7: Real-Time Prediction
- Integrate the model with a real-time data pipeline to make live predictions.
python
def predict_traffic(real_time_data):
input_features = real_time_data[[‘hour’, ‘day_of_week’, ‘lag_1’, ‘rolling_avg’]]
prediction = model.predict(input_features)
return prediction
Step 8: Visualization and Deployment (Optional)
- Build a dashboard using Streamlit or Tableau to display traffic predictions.
python
import streamlit as st
st.title(“Real-Time Traffic Flow Prediction”)
user_hour = st.slider(“Select Hour of Day:”, 0, 23, 8)
predicted_flow = model.predict([[user_hour, 1, 50, 48]]) # Example input
st.write(f”Predicted Traffic Flow: {predicted_flow[0]:.2f}”)
Expected Outcomes
- A model capable of accurately predicting traffic flow based on real-time and historical data.
- Insights into patterns and factors influencing traffic congestion.
- Hands-on experience with time-series analysis and real-time data handling.
- An interactive dashboard for stakeholders to access traffic forecasts.
Project 6:E-Commerce Product Recommendation System In Data Science
Project Description
This Data Science projects focuses on building a recommendation system for an e-commerce platform to enhance user experience and boost sales. The system uses collaborative filtering, content-based filtering, and hybrid approaches to recommend products tailored to individual user preferences. By analyzing user purchase history, product attributes, and browsing behavior, the recommendation system provides personalized suggestions. This hands-on project equips you with knowledge of recommendation algorithms and their real-world application in e-commerce.
Skills Required
- Data Analysis and Manipulation:
- Proficiency in Python with libraries like Pandas and NumPy.
- Machine Learning for Recommendations:
- Understanding of collaborative and content-based filtering techniques.
- Matrix Factorization Techniques:
- Familiarity with SVD, ALS, or other advanced recommendation methods.
- Visualization:
- Expertise in Matplotlib, Seaborn, or Plotly for insights.
- Evaluation Metrics:
- Knowledge of evaluation metrics such as RMSE, Precision, Recall, and F1-Score.
Steps to Execute the Project
Step 1: Data Collection
- Use publicly available datasets such as the Amazon Product Dataset or MovieLens Dataset for training and evaluation.
python
import pandas as pd
# Load dataset
data = pd.read_csv(‘ecommerce_data.csv’) # Replace with actual dataset
print(data.head())
Step 2: Exploratory Data Analysis (EDA)
- Analyze user-product interactions and visualize purchase patterns.
python
import seaborn as sns
import matplotlib.pyplot as plt
# Plotting most purchased products
top_products = data[‘product_id’].value_counts().head(10)
sns.barplot(x=top_products.index, y=top_products.values)
plt.title(‘Top 10 Most Purchased Products’)
plt.xlabel(‘Product ID’)
plt.ylabel(‘Number of Purchases’)
plt.show()
Step 3: Preprocessing
- Prepare data by handling missing values and creating user-item interaction matrices.
python
# Creating a user-item interaction matrix
interaction_matrix = data.pivot_table(index=’user_id’, columns=’product_id’, values=’rating’, fill_value=0)
Step 4: Build the Recommendation Model
Collaborative Filtering
- Use matrix factorization techniques like Singular Value Decomposition (SVD).
python
from surprise import SVD, Dataset, Reader
from surprise.model_selection import train_test_split
from surprise import accuracy
# Prepare data for Surprise library
reader = Reader(rating_scale=(1, 5))
dataset = Dataset.load_from_df(data[[‘user_id’, ‘product_id’, ‘rating’]], reader)
trainset, testset = train_test_split(dataset, test_size=0.2)
# Train the model
model = SVD()
model.fit(trainset)
# Evaluate the model
predictions = model.test(testset)
rmse = accuracy.rmse(predictions)
print(f”RMSE: {rmse}”)
Content-Based Filtering
- Recommend products based on product attributes like category, brand, or features.
python
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import linear_kernel
# Create a TF-IDF matrix for product descriptions
tfidf = TfidfVectorizer(stop_words=’english’)
tfidf_matrix = tfidf.fit_transform(data[‘product_description’])
# Calculate cosine similarity
cosine_sim = linear_kernel(tfidf_matrix, tfidf_matrix)
Hybrid Approach
- Combine collaborative and content-based filtering for enhanced recommendations.
python
# Weighted average of collaborative and content-based scores
def hybrid_recommend(user_id, product_id, alpha=0.7):
collab_score = model.predict(user_id, product_id).est
content_score = cosine_sim[product_id, :].mean()
return alpha * collab_score + (1 – alpha) * content_score
Step 5: Deployment
- Build a dashboard or API to recommend products to users dynamically.
python
import streamlit as st
st.title(“E-Commerce Product Recommendation System”)
user_id = st.text_input(“Enter User ID:”)
if user_id:
recommended_products = [1, 2, 3] # Replace with model recommendations
st.write(f”Recommended Products for User {user_id}: {recommended_products}”)
Expected Outcomes
- A fully functional recommendation system for personalized product suggestions.
- Insights into user behavior and product popularity.
- Practical knowledge of collaborative and content-based filtering techniques.
- Improved user engagement and potential for increased sales in an e-commerce platform.
Project 7:Credit Card Fraud Detection Using Machine Learning In Data Science
Project Description
The goal of this Data Science project is to detect fraudulent credit card transactions using machine learning techniques. By analyzing transaction data, the system identifies patterns and anomalies that indicate potential fraud. The project involves processing imbalanced datasets, implementing classification models, and evaluating their performance using various metrics. Fraud detection systems are crucial for ensuring secure financial transactions and minimizing economic losses for organizations and individuals.
Skills Required
- Data Preprocessing:
- Handling imbalanced datasets using techniques like SMOTE or undersampling.
- Machine Learning:
- Knowledge of algorithms like Logistic Regression, Random Forest, Gradient Boosting, or Neural Networks.
- Evaluation Metrics:
- Familiarity with metrics like Precision, Recall, F1-Score, and AUC-ROC.
- Feature Engineering:
- Creating meaningful features from raw data for better model performance.
- Programming and Libraries:
- Expertise in Python, Pandas, NumPy, Scikit-learn, and Matplotlib/Seaborn.
Steps to Execute the Project
Step 1: Data Collection
- Use publicly available datasets like the Kaggle Credit Card Fraud Dataset.
python
import pandas as pd
# Load dataset
data = pd.read_csv(“creditcard.csv”)
print(data.head())
Step 2: Exploratory Data Analysis (EDA)
- Analyze the class distribution, transaction amounts, and feature correlations.
- Visualize the imbalance between fraudulent and non-fraudulent transactions.
python
import seaborn as sns
import matplotlib.pyplot as plt
# Class distribution
sns.countplot(x=’Class’, data=data)
plt.title(“Class Distribution (0: Legit, 1: Fraud)”)
plt.show()
# Correlation heatmap
sns.heatmap(data.corr(), cmap=’coolwarm’, annot=False)
plt.title(“Feature Correlation”)
plt.show()
Step 3: Data Preprocessing
- Handle class imbalance using SMOTE or undersampling.
- Normalize continuous features for model input.
python
from sklearn.model_selection import train_test_split
from imblearn.over_sampling import SMOTE
# Split data into features and target
X = data.drop(‘Class’, axis=1)
y = data[‘Class’]
# Handle imbalance with SMOTE
smote = SMOTE(random_state=42)
X_resampled, y_resampled = smote.fit_resample(X, y)
# Split into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X_resampled, y_resampled, test_size=0.2, random_state=42)
Step 4: Model Selection and Training
- Train multiple models like Logistic Regression, Random Forest, and Gradient Boosting.
- Tune hyperparameters for optimal performance.
python
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import classification_report, roc_auc_score
# Train Random Forest model
model = RandomForestClassifier(random_state=42)
model.fit(X_train, y_train)
# Evaluate model
y_pred = model.predict(X_test)
print(classification_report(y_test, y_pred))
print(“ROC-AUC Score:”, roc_auc_score(y_test, y_pred))
Step 5: Model Evaluation
- Use metrics like Precision, Recall, F1-Score, and AUC-ROC to assess performance.
- Generate a confusion matrix to analyze false positives and negatives.
python
from sklearn.metrics import confusion_matrix, ConfusionMatrixDisplay
# Confusion matrix
cm = confusion_matrix(y_test, y_pred)
disp = ConfusionMatrixDisplay(confusion_matrix=cm, display_labels=[“Legit”, “Fraud”])
disp.plot()
plt.show()
Step 6: Deploying the Model
- Save the trained model and deploy it using Flask or Streamlit for real-time fraud detection.
python
import pickle
# Save the model
with open(‘fraud_detection_model.pkl’, ‘wb’) as file:
pickle.dump(model, file)
# Example of loading and predicting
with open(‘fraud_detection_model.pkl’, ‘rb’) as file:
loaded_model = pickle.load(file)
# Predict on new data
new_data = X_test.iloc[0:1]
print(“Prediction:”, loaded_model.predict(new_data))
Expected Outcomes
- A machine learning model designed to identify fraudulent credit card transactions.
- Insights into transaction patterns and behaviors associated with fraud.
- Hands-on experience with handling imbalanced datasets and classification problems.
- Improved understanding of evaluation metrics in binary classification.
Project 8:Personalized Health Risk Prediction Using Public Health Datasets In Data Science
Project Description
This Data Science project focuses on predicting personalized health risks by analyzing public health datasets. Using machine learning, the system identifies potential health conditions or risks based on individual data like age, gender, lifestyle habits, and medical history. Public health datasets such as those from the CDC or WHO provide valuable insights into risk factors for chronic diseases, mental health issues, or infectious diseases. The primary goal is to enable early intervention and promote better health outcomes through tailored recommendations.
Skills Required
- Data Analysis:
- Proficiency in Python for data cleaning and analysis using Pandas and NumPy.
- Machine Learning:
- Expertise in classification models like Logistic Regression, Random Forest, and XGBoost.
- Data Visualization:
- Knowledge of visualization libraries like Seaborn, Matplotlib, and Plotly.
- Domain Knowledge:
- Understanding of health risk factors and epidemiological concepts.
- Feature Engineering:
- Ability to derive meaningful features from raw data for improved predictions.
Steps to Execute the Project
Step 1: Data Collection
- Utilize public health datasets from platforms like:
- CDC (Centers for Disease Control and Prevention)
- WHO (World Health Organization)
- Kaggle (e.g., Heart Disease or Diabetes datasets)
python
import pandas as pd
# Load dataset
data = pd.read_csv(“health_dataset.csv”) # Replace with actual dataset
print(data.head())
Step 2: Data Cleaning and Preprocessing
- Handle missing values, normalize continuous variables, and encode categorical features.
python
# Fill missing values
data.fillna(data.mean(), inplace=True)
# Encoding categorical variables
data = pd.get_dummies(data, columns=[‘Gender’, ‘Smoking_Status’], drop_first=True)
Step 3: Exploratory Data Analysis (EDA)
- Analyze the relationship between health metrics and risk factors.
- Visualize distributions and correlations.
python
import seaborn as sns
import matplotlib.pyplot as plt
# Correlation heatmap
sns.heatmap(data.corr(), annot=True, cmap=’coolwarm’)
plt.title(“Feature Correlation”)
plt.show()
# Distribution of health risks
sns.countplot(x=’Health_Risk’, data=data)
plt.title(“Health Risk Distribution”)
plt.show()
Step 4: Feature Engineering
- Create composite features like BMI, risk scores, or age group categories.
python
# Calculate BMI (Body Mass Index)
data[‘BMI’] = data[‘Weight_kg’] / (data[‘Height_m’] ** 2)
# Create age groups
data[‘Age_Group’] = pd.cut(data[‘Age’], bins=[0, 30, 50, 70, 100], labels=[‘Youth’, ‘Adult’, ‘Senior’, ‘Elder’])
Step 5: Model Building
- Train machine learning models like Logistic Regression, Random Forest, or XGBoost.
- Split the data into training and testing sets.
python
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import classification_report
# Split data
X = data.drop(‘Health_Risk’, axis=1)
y = data[‘Health_Risk’]
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train model
model = RandomForestClassifier(random_state=42)
model.fit(X_train, y_train)
# Evaluate model
y_pred = model.predict(X_test)
print(classification_report(y_test, y_pred))
Step 6: Model Evaluation
- Evaluate using metrics like Accuracy, Precision, Recall, and ROC-AUC.
- Generate a confusion matrix to analyze predictions.
python
from sklearn.metrics import confusion_matrix, roc_auc_score
# Confusion Matrix
cm = confusion_matrix(y_test, y_pred)
print(“Confusion Matrix:\n”, cm)
# ROC-AUC Score
roc_auc = roc_auc_score(y_test, model.predict_proba(X_test)[:, 1])
print(f”ROC-AUC Score: {roc_auc}”)
Step 7: Deployment
- Build a web application or API for users to input data and receive health risk predictions.
- Use frameworks like Flask or Streamlit.
python
import streamlit as st
st.title(“Personalized Health Risk Prediction”)
age = st.number_input(“Enter Age:”)
gender = st.selectbox(“Select Gender:”, [“Male”, “Female”])
smoking_status = st.selectbox(“Smoking Status:”, [“Non-Smoker”, “Smoker”])
bmi = st.number_input(“Enter BMI:”)
# Example prediction
if st.button(“Predict Health Risk”):
prediction = model.predict([[age, gender == “Male”, smoking_status == “Smoker”, bmi]])
st.write(f”Predicted Health Risk: {‘High’ if prediction[0] else ‘Low’}”)
Expected Outcomes
- A predictive model capable of identifying individual health risks.
- Insights into factors contributing to specific health conditions.
- Hands-on experience with public health data and developing risk prediction models.
- An interactive tool for users to assess their health risks and receive recommendations.
Project 9:Social Media Engagement Analysis and Sentiment Prediction In Data Science
Project Description
This Data Science project aims to analyze social media engagement and predict the sentiment of posts using natural language processing (NLP) and machine learning techniques. By analyzing text data from platforms like Twitter, Instagram, or Facebook, this system identifies how users are interacting with posts and classifies the sentiment into categories like positive, negative, or neutral. The insights gained from sentiment analysis can help brands, marketers, and businesses understand public opinion, improve customer engagement, and enhance content strategies.
Skills Required
- Text Preprocessing and NLP:
- Expertise in text cleaning, tokenization, stemming, and lemmatization.
- Machine Learning:
- Knowledge of classification algorithms like Logistic Regression, Random Forest, and Naive Bayes.
- Natural Language Processing (NLP):
- Proficiency in libraries like NLTK, SpaCy, or Hugging Face Transformers.
- Data Analysis and Visualization:
- Familiarity with Pandas, Matplotlib, Seaborn, and Plotly.
- Sentiment Analysis:
- Understanding of sentiment analysis and text classification models.
Steps to Execute the Project
Step 1: Data Collection
- Gather social media data using APIs (e.g., Twitter API, Facebook Graph API) or use publicly available datasets.
python
import tweepy
# Set up the API client (you need to have your own credentials)
consumer_key = “your_consumer_key”
consumer_secret = “your_consumer_secret”
access_token = “your_access_token”
access_token_secret = “your_access_token_secret”
auth = tweepy.OAuth1UserHandler(consumer_key, consumer_secret, access_token, access_token_secret)
api = tweepy.API(auth)
# Collect tweets based on a keyword or hashtag
tweets = api.search_tweets(q=’#socialmedia’, count=100, lang=’en’)
# Extract tweet texts
tweet_texts = [tweet.text for tweet in tweets]
Step 2: Data Preprocessing
- Clean the data by removing stopwords, special characters, URLs, and hashtags.
python
import re
from nltk.corpus import stopwords
# Preprocessing function to clean tweet text
def clean_text(text):
text = re.sub(r’http\S+’, ”, text) # Remove URLs
text = re.sub(r’@\w+’, ”, text) # Remove mentions
text = re.sub(r’#[A-Za-z0-9]+’, ”, text) # Remove hashtags
text = re.sub(r'[^A-Za-z0-9\s]’, ”, text) # Remove special characters
text = text.lower() # Convert to lowercase
text = ‘ ‘.join([word for word in text.split() if word not in stopwords.words(‘english’)]) # Remove stopwords
return text
# Apply cleaning function
cleaned_tweets = [clean_text(tweet) for tweet in tweet_texts]
Step 3: Feature Extraction
- Convert text data into numerical features using techniques like TF-IDF or word embeddings.
python
from sklearn.feature_extraction.text import TfidfVectorizer
# TF-IDF vectorization
vectorizer = TfidfVectorizer(max_features=5000)
X = vectorizer.fit_transform(cleaned_tweets).toarray()
Step 4: Sentiment Labeling
- Label the sentiment of the tweets (positive, negative, neutral) either manually or using a pre-labeled dataset.
python
# Example sentiment labels (this would normally come from a labeled dataset)
y = [‘positive’, ‘negative’, ‘neutral’, ‘positive’, ‘negative’] # Example labels
Step 5: Model Building and Training
- Train a machine learning model (Logistic Regression, Naive Bayes, or Random Forest) to predict sentiment.
python
from sklearn.model_selection import train_test_split
from sklearn.naive_bayes import MultinomialNB
from sklearn.metrics import classification_report, accuracy_score
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a Naive Bayes model
model = MultinomialNB()
model.fit(X_train, y_train)
# Evaluate the model
y_pred = model.predict(X_test)
print(classification_report(y_test, y_pred))
Step 6: Social Media Engagement Analysis
- Analyze user engagement metrics like likes, shares, comments, and followers to gauge content success.
- Correlate sentiment with engagement metrics to understand the impact of sentiment on user interaction.
python
import matplotlib.pyplot as plt
# Example engagement data (likes, shares, comments)
engagement_data = {‘likes’: [100, 150, 200, 50, 120], ‘shares’: [10, 15, 20, 5, 12], ‘comments’: [5, 7, 3, 2, 6]}
# Create a DataFrame for analysis
engagement_df = pd.DataFrame(engagement_data)
# Visualize engagement distribution
plt.figure(figsize=(10, 5))
plt.bar(engagement_df.index, engagement_df[‘likes’], color=’blue’, label=’Likes’)
plt.bar(engagement_df.index, engagement_df[‘shares’], bottom=engagement_df[‘likes’], color=’green’, label=’Shares’)
plt.bar(engagement_df.index, engagement_df[‘comments’], bottom=engagement_df[‘likes’] + engagement_df[‘shares’], color=’red’, label=’Comments’)
plt.xlabel(‘Posts’)
plt.ylabel(‘Engagement’)
plt.title(‘Social Media Engagement Analysis’)
plt.legend()
plt.show()
Step 7: Sentiment Prediction and Engagement Impact
- Use the trained model to predict sentiment for new social media posts and analyze their engagement potential.
- Implement a recommendation system for improving engagement based on sentiment trends.
python
# Predict sentiment for a new post
new_post = “This is a fantastic product!”
new_post_cleaned = clean_text(new_post)
new_post_vectorized = vectorizer.transform([new_post_cleaned]).toarray()
sentiment = model.predict(new_post_vectorized)
print(f”Predicted Sentiment: {sentiment[0]}”)
Expected Outcomes
- A sentiment analysis model capable of classifying social media posts as positive, negative, or neutral.
- Insights into how sentiment influences social media engagement.
- Visualizations and reports showcasing content performance across different sentiment categories.
- Real-time sentiment prediction for new social media posts and recommendations for engagement strategies.

Project 10:Energy Consumption Forecasting with Time-Series Analysis In Data Science
Project Description
This Data Science project involves forecasting energy consumption using time-series analysis techniques. The goal is to predict future energy usage based on historical consumption data. Accurate energy consumption forecasts are crucial for optimizing energy production, reducing waste, and planning infrastructure. Time-series forecasting methods like ARIMA, SARIMA, and LSTM (Long Short-Term Memory) models will be applied to historical energy usage data to predict future demand. This project will demonstrate how predictive models can be leveraged to make data-driven decisions in energy management.
Skills Required
- Time-Series Analysis:
- Proficiency in time-series forecasting techniques like ARIMA, SARIMA, and exponential smoothing.
- Machine Learning:
- Familiarity with machine learning models, particularly LSTM networks for time-series forecasting.
- Data Analysis:
- Skilled in using Python libraries like Pandas and NumPy for data manipulation.
- Visualization:
- Familiarity with Matplotlib and Seaborn for visualizing time-series data and extracting insights.
- Forecasting and Evaluation:
- Experience in evaluating model accuracy using metrics like RMSE, MAE, and MAPE.
Steps to Execute the Project
Step 1: Data Collection
- Collect historical energy consumption data from publicly available sources or use datasets like the UCI Machine Learning Repository or Kaggle.
- The dataset should include hourly, daily, or monthly energy usage, along with any relevant external factors (e.g., temperature, holidays, or industrial activity).
python
import pandas as pd
# Load energy consumption dataset
data = pd.read_csv(‘energy_consumption.csv’, parse_dates=[‘Date’], index_col=’Date’)
print(data.head())
Step 2: Data Preprocessing
- Handle missing values, convert date columns to datetime objects, and ensure data is properly formatted for time-series analysis.
python
# Fill missing values with forward fill method
data.fillna(method=’ffill’, inplace=True)
# Check for missing data and outliers
data.plot()
Step 3: Exploratory Data Analysis (EDA)
- Visualize energy consumption patterns over time.
- Decompose the time-series data to understand trends, seasonality, and noise.
python
import matplotlib.pyplot as plt
from statsmodels.tsa.seasonal import seasonal_decompose
# Decompose the time series
decomposed = seasonal_decompose(data[‘Consumption’], model=’additive’, period=365)
decomposed.plot()
plt.show()
# Visualize the energy consumption over time
data[‘Consumption’].plot(figsize=(12, 6))
plt.title(“Energy Consumption Over Time”)
plt.xlabel(“Date”)
plt.ylabel(“Consumption”)
plt.show()
Step 4: Time-Series Forecasting with ARIMA
- Utilize ARIMA (AutoRegressive Integrated Moving Average) to model and analyze time series data.
- Determine the optimal parameters (p, d, q) using techniques like ACF (AutoCorrelation Function) and PACF (Partial AutoCorrelation Function).
python
from statsmodels.tsa.arima.model import ARIMA
# Train-test split (80-20%)
train_data, test_data = data[‘Consumption’][:int(0.8*len(data))], data[‘Consumption’][int(0.8*len(data)):]
# Fit ARIMA model (p=1, d=1, q=1 as an example)
model = ARIMA(train_data, order=(1, 1, 1))
model_fit = model.fit()
# Forecast on test data
forecast = model_fit.forecast(steps=len(test_data))
plt.plot(test_data.index, test_data, label=’Actual’)
plt.plot(test_data.index, forecast, label=’Forecasted’)
plt.legend()
plt.title(“ARIMA Forecasting: Energy Consumption”)
plt.show()
Step 5: Model Evaluation
- Evaluate the model performance using error metrics such as Root Mean Squared Error (RMSE), Mean Absolute Error (MAE), and Mean Absolute Percentage Error (MAPE).
python
from sklearn.metrics import mean_squared_error, mean_absolute_error
import numpy as np
# Evaluate using RMSE and MAE
rmse = np.sqrt(mean_squared_error(test_data, forecast))
mae = mean_absolute_error(test_data, forecast)
print(f”RMSE: {rmse}, MAE: {mae}”)
Step 6: Advanced Model – LSTM (Long Short-Term Memory)
- Implement LSTM, a type of neural network designed for time-series forecasting.
- Preprocess data for LSTM input, which requires reshaping the data into sequences.
python
from keras.models import Sequential
from keras.layers import LSTM, Dense
from sklearn.preprocessing import MinMaxScaler
# Scale the data
scaler = MinMaxScaler(feature_range=(0, 1))
scaled_data = scaler.fit_transform(data[‘Consumption’].values.reshape(-1, 1))
# Prepare data for LSTM model (creating sequences)
X, y = [], []
for i in range(60, len(scaled_data)):
X.append(scaled_data[i-60:i, 0])
y.append(scaled_data[i, 0])
X = np.array(X)
y = np.array(y)
# Reshape data to be compatible with LSTM input
X = np.reshape(X, (X.shape[0], X.shape[1], 1))
# Build the LSTM model
model = Sequential()
model.add(LSTM(units=50, return_sequences=True, input_shape=(X.shape[1], 1)))
model.add(LSTM(units=50))
model.add(Dense(units=1))
model.compile(optimizer=’adam’, loss=’mean_squared_error’)
# Train the model
model.fit(X, y, epochs=10, batch_size=32)
# Predict the future values
predicted_consumption = model.predict(X)
predicted_consumption = scaler.inverse_transform(predicted_consumption)
# Plot predictions vs actual consumption
plt.plot(data.index[60:], data[‘Consumption’][60:], label=’Actual’)
plt.plot(data.index[60:], predicted_consumption, label=’Predicted’)
plt.legend()
plt.title(“LSTM Forecasting: Energy Consumption”)
plt.show()
Step 7: Forecasting Future Energy Consumption
- Forecast energy consumption for the next 30 days or longer based on the trained model.
python
# Forecast future energy consumption using ARIMA
future_forecast = model_fit.forecast(steps=30)
future_dates = pd.date_range(start=data.index[-1], periods=30, freq=’D’)
plt.plot(future_dates, future_forecast, label=’Forecasted Future Consumption’, color=’red’)
plt.legend()
plt.title(“Future Energy Consumption Forecasting (ARIMA)”)
plt.show()
Expected Outcomes
- A time-series forecasting model (ARIMA or LSTM) capable of predicting future energy consumption.
- Visualization of historical energy consumption trends, seasonal patterns, and forecast accuracy.
- Model performance evaluation using error metrics like RMSE, MAE, and MAPE.
- Practical experience in handling time-series data and forecasting using classical and deep learning methods.
Conclusion:
To expand further, data science projects for beginners are essential stepping stones in developing a well-rounded understanding of the field. They provide exposure to various aspects of the data science pipeline, from data collection and cleaning to model building and deployment. By taking on these Data Science projects for beginners can also explore different machine learning algorithms, data visualization techniques, and statistical methods, while gaining a deeper understanding of data interpretation.
Additionally, these Data Science projects For Beginners serve as a powerful tool for building a strong portfolio, showcasing problem-solving abilities and technical skills to potential employers or clients. They offer valuable insight into industry-specific challenges, making beginners more marketable and well-prepared for more complex tasks. The hands-on experience gained through data science projects builds confidence, encourages self-learning, and fosters a deeper passion for exploring data-driven solutions.
Overall, undertaking data science projects early on in one’s career or learning journey not only accelerates skill development but also opens the door to endless opportunities in the ever-expanding field of data science.
Frequently Asked Questions (FAQS) For Data Science Projects For Beginners
What are some recommended data science projects for beginners?
Some great beginner projects include predictive modeling, data cleaning, exploratory data analysis (EDA), and simple machine learning models. Projects like predicting house prices, analyzing customer churn, or visualizing data can be good starting points.
How do I choose a data science project for beginners?
When selecting a project, pick a dataset you’re interested in and ensure it’s not too complex. Start with something that allows you to practice basic concepts like data cleaning, visualization, and model building.
What tools are essential for beginners working on data science projects?
Important tools for beginners include Python, Jupyter Notebooks, libraries such as Pandas, NumPy, Matplotlib, and Scikit-learn, as well as environments like Anaconda for managing packages.
How can beginners develop their data science skills by working on projects?
By working on projects, beginners can gain hands-on experience in data collection, cleaning, visualization, and modeling. This enables them to implement theoretical concepts in real-world situations.
Are there any open-source datasets for beginners to practice data science?
Yes, platforms like Kaggle, UCI Machine Learning Repository, and data.gov offer open-source datasets that are perfect for beginners.
What is the typical workflow for a beginner data science project?
The typical workflow includes problem definition, data collection, data cleaning, exploratory data analysis (EDA), feature engineering, model building, evaluation, and presentation of results.
Can I use data science projects to build a portfolio?
Absolutely! Showcasing your data science projects, including the steps you took and the results you achieved, will make your portfolio stand out to potential employers or clients.
How do I get started with a data science project as a beginner?
Start by choosing a project that matches your skill level, find a relevant dataset, clean and preprocess the data, explore the data using visualizations, and build a simple model to make predictions or insights.
What challenges should beginners expect in data science projects?
Beginners might face challenges in data cleaning, choosing the right model, and evaluating the model’s performance. However, overcoming these challenges will enhance your learning and skills.
How long does it take to complete a beginner-level data science project?
The time to complete a beginner project depends on its complexity, but generally, it can take anywhere from a few hours to a couple of weeks to finish, especially if you’re learning along the way.